10 Tips To Write A Secure Javascript Code
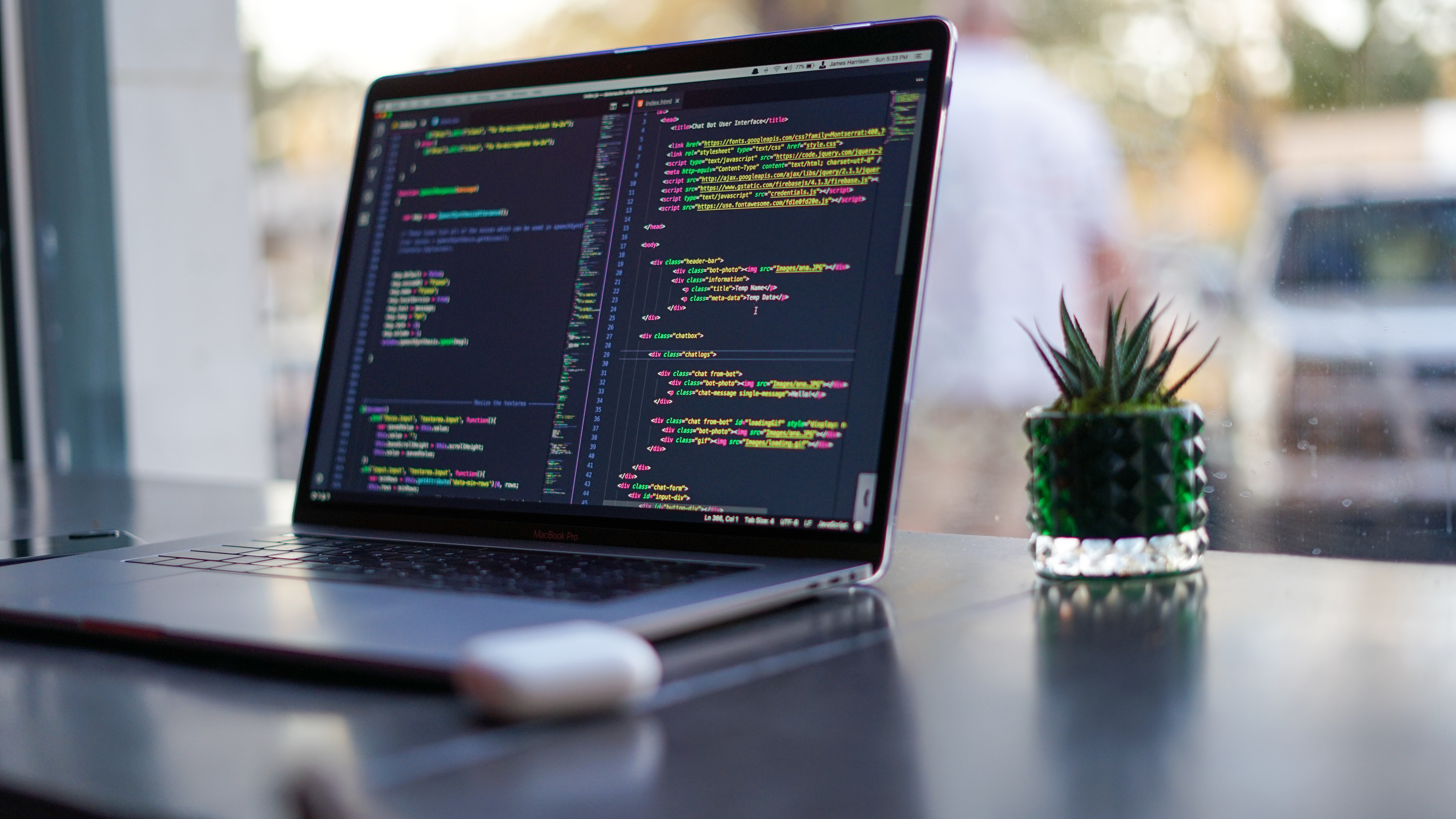
Writing secure JavaScript code is needed to make secure web applications. Writing secure JavaScript code is important for protecting user data, stopping security holes, keeping trust and credibility, following rules, avoiding downtime and financial losses, and keeping trust and credibility. Here are some ways to make sure that your JavaScript code is safe:
1. Validate user input
Don’t trust what a user puts in; check it every time. Attackers can change what users type, and validating it can stop injection attacks and other flaws. Here’s an example of JavaScript code that checks if the email address entered by the user is valid or not:
In this case, the validateEmail() function uses a regular expression to check the input to see if it looks like a valid email address. The test() method returns true if the input matches the pattern; otherwise, it returns false.
The code then uses the prompt() method to ask the user for their email address and stores it in the userInput variable. After the validateEmail() function checks that the email address in the input is correct, the alert() method shows the right message.
2. Set mode to strict
If you turn on the strict mode in your JavaScript code, you can avoid making common programming mistakes and lower the risk of security holes. For example, if you want strict mode to be turned on for your whole script, you can add the following code to the top of your file:
By enforcing stricter syntax and semantics, a strict mode will help you avoid making common programming mistakes and make your code safer. For example, the strict mode will stop you from using variables that haven’t been declared, from deleting properties that can’t be deleted, and from using the “eval” function in a dangerous way.
3. Avoid global variables
Attackers can’t change your code or get sensitive information if you use local variables. Here’s an example of how you can use local variables instead of global variables in your JavaScript code:
In the above example, we created a “secretData” local variable inside the “myFunction” function. This means that the variable can only be accessed within the scope of the function. No other part of the code, including an attacker, can access or change the variable. By putting sensitive data in local variables, you can stop attackers from seeing or changing that data.
On the other hand, if we had made “secretData” a global variable, it would have looked like this:
Any part of the code could access the “secretData” variable, so an attacker could potentially change its value or read its contents. This is dangerous and should be avoided as much as possible.
4. Use HTTPS
Always use HTTPS to keep communication between clients and servers safe. Attackers won’t be able to steal sensitive information or change how people talk. By using HTTPS, you can stop attackers from listening in on the conversation or changing the data that is being sent. Here’s how to use HTTPS to communicate between a client and a server:
In the above example, we send a GET request to the “/myapi” endpoint on the “example.com” server using the “https” module that comes with Node.js. The hostname, port, path, and method for the request are all set by the options object.
By using HTTPS instead of HTTP, the communication between the client and server is encrypted. This means that anyone trying to intercept or change the data being sent won’t be able to read or change the data. This helps keep private information safe and stops attacks like eavesdropping, “man-in-the-middle” attacks, and changing data.
5. Sanitize data
To stop cross-site scripting (XSS) attacks, clean up data before showing it to the user. Use HTML escaping or encoding to stop scripts that are bad from running. XSS attacks happen when a hacker puts malicious code into a web page. This code can then be run in the browsers of users who visit the page without knowing it. Here’s how this can be done with HTML escaping:
In the above example, the escapeHtml function takes a string as input and replaces any special characters that could be used in an XSS attack with their HTML entities. For example, the less-than sign (“”) is replaced with “”, which is the HTML entity for the less-than sign.
By sanitizing user input in this way, you can stop malicious scripts from running in the browser. This keeps your users’ data and devices safe from harm.
6. Avoid eval ()
Don’t use the eval() function because it can run any code and can lead to security holes. If an attacker is able to add malicious code to the input, they can run that code on the user’s device, which could compromise their data and security. To avoid this risk, it’s usually best to use other methods for running code dynamically, such as the Function() constructor, or to avoid running code dynamically whenever possible. Here’s an example of how the Function() constructor can be used to run code that changes over time:
In the above example, the user is asked to enter a number, which is then used to create a string of dynamic code that multiplies the number by 2. Then, the Function() constructor is used to make a new function that runs the code when it is called.
If you use the Function() constructor instead of eval(), you can run code dynamically and reduce the security risks that come with eval (). But it’s important to remember that using the Function() constructor still has some risks because an attacker can use it to run any code they want. So, it’s still important to properly validate and clean any user input that is used to make dynamic code.
7. Use Policy for Content Security (CSP)
Use CSP to limit the resources your web application can load and stop cross-site scripting (XSS) attacks and other vulnerabilities. Here’s one way you can use the Content Security Policy (CSP) to protect your web application from XSS attacks:
Content-Security-Policy: default-src ‘self’; script-src ‘self’ https://trustedcdn.example.com;
In this example, we are using CSP to set a policy that limits the resources that our web application can load. The default-src directive says that resources should only be loaded from the same origin as the web application itself. The script-src directive says that scripts can be loaded from the same origin as the web application or from https://trustedcdn.example.com, which is a trusted CDN.
By setting this policy, we stop any scripts or resources from being loaded from outside sources that aren’t explicitly allowed. This means that if an attacker tries to inject a malicious script from the outside into our web application, CSP will block the script, stopping the XSS attack.
It’s important to remember that implementing CSP alone might not be enough to fully protect your web application from XSS attacks. It’s still important to follow other security best practices like input validation, sanitization, and encoding to make sure that your web application is fully protected against vulnerabilities.
8. Be careful when using libraries from outside your company
Be careful when using third-party libraries in your JavaScript code. Make sure they come from a trustworthy source and have the latest security updates. Here’s an example of how you could use “DatabaseUtils” in your JavaScript code with these safety measures in place:
By taking these precautions, you can be sure to use third-party libraries in your JavaScript code carefully and lower the risk of security holes in your web application.
9. Use password hashing and encryption
Password hashing is a method that uses a one-way algorithm to turn a password into a hash value. This makes it hard for an attacker to use the hash value to figure out the original password. Hash passwords with a secure algorithm like bcrypt or Argon2. Here’s an example of how to use bcrypt in Node to hash a password. js
Use encryption. Encryption is a method that uses a secret key to turn plain text into cipher text. To protect sensitive data, use a secure encryption algorithm like AES or RSA. Here’s an example of how to use AES encryption in Node.js to protect a sensitive string:
Don’t store passwords in plain text: It’s important not to store passwords in plain text because that makes it easy for an attacker to get them. Instead, store the user’s hashed password and compare it to the user’s hashed password when authenticating. Here’s an example of how to use bcrypt in Node.js to compare a hashed password with a user’s input:
By following these steps, you can use password hashing and encryption to protect sensitive data in your web application and reduce the risk of data breaches and security holes.
10. Make sure your browser has safety features
Use security features like SameSite cookies, HTTP-only cookies, and Cross-Origin Resource Sharing in your browser to stop attacks (CORS). Here are some examples of security features you can use to stop attacks on your web application:
SameSite cookies are used to stop CSRF attacks by making them so that they can only be used on the site that created them. This makes it harder for attackers to steal a user’s session data or take unauthorized actions on the user’s behalf. Here’s an example of how to use JavaScript to set a SameSite cookie:
Only work with HTTP: By making it impossible for JavaScript code to read or change the cookie, HTTP-only cookies can be used to stop XSS attacks. This makes it harder for attackers to steal a user’s session data or take unauthorized actions on the user’s behalf. Here’s how to set a cookie that works only with HTTP in JavaScript:
Cross-Origin Resource Sharing (CORS): CORS limits cross-domain requests to stop unauthorized access to a web server’s resources. This makes it harder for attackers to get to sensitive data or take actions without the user’s permission. Here’s how CORS headers can be set on a web server:
Access-Control-Allow-Origin: https://www.example.com
Access-Control-Allow-Methods: GET, POST, OPTIONS
Access-Control-Allow-Headers: Content-Type
Some of the best ways to write safe JavaScript code are listed here. You can, however, make your web application safer in many other ways. Keep up with the latest best practices and trends in security to stay ahead of attackers. Moreover, there’s a bigger topic of operationalizing secure coding as part of the way development teams work and their coding guidelines. It takes experience to develop and establish this and our company can help you.
JWay Group, Inc is an IT, digital, and staffing company with more than 25 years of experience. We use Javascript/Typescript for full-stack and backend development for the many projects we’ve worked with. If you are interested in learning more about how we can help you establish your team and its secure coding guidelines, or perhaps you are in need of Javascript/Typescript developers for your project, feel free to contact us or send an email to info@jway.com.